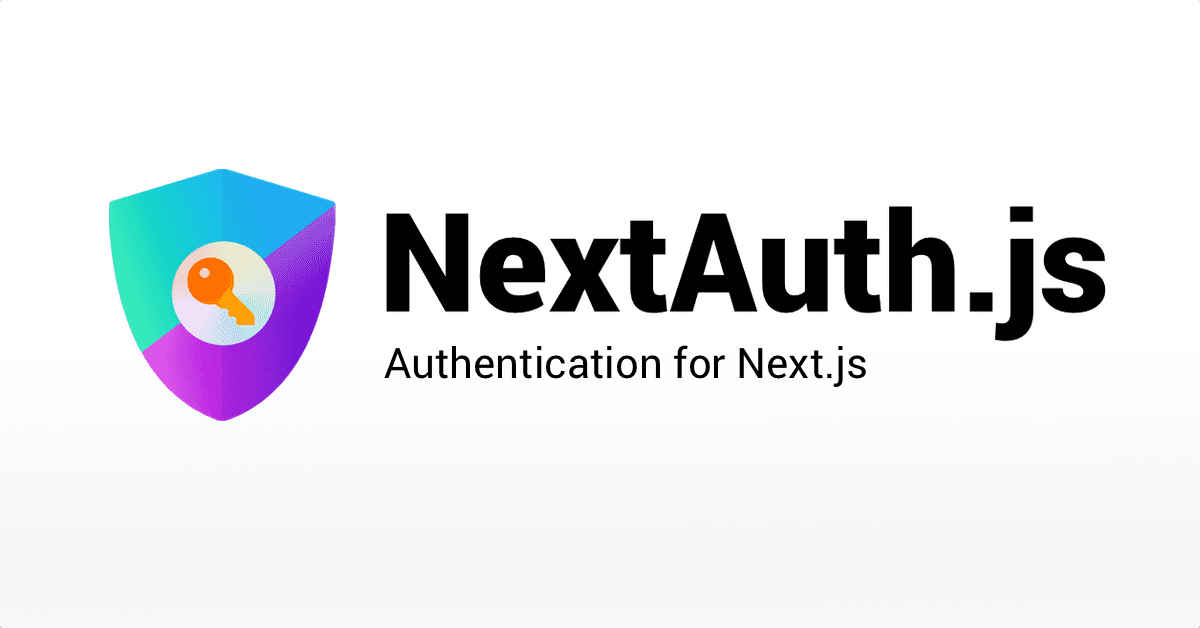
NextJS 14 Social Login with NextAuth
In this article I will describe the step by step process how I got a user with a github account to login in a NextJS app.
Step1: Initialise a NextJS project and Install NextAuth
we will be using the bleeding edge NextAuth version 5 which is in beta release. at the time of writing this blog NextAuth is currently on version 4 in the documentation here
> npm install next-auth:5.0.0-beta.19
Step 2: create a auth.ts file inside a lib folder
import NextAuth from 'next-auth';
import Facebook from 'next-auth/providers/facebook';
import GitHub from "next-auth/providers/github"
import Google from "next-auth/providers/google"
export const { handlers, signIn, signOut, auth } = NextAuth({
providers: [Facebook, GitHub, Google]
});
Step 3: Add your credentials to .env.local
AUTH_GITHUB_ID='XXXXXXXXXXXXX'
AUTH_GITHUB_SECRET='XXXXXXXXXXXXX'
AUTH_SECRET='XXXXXXXXXXXXX'
You will need to login to Github and Facebook and create these credentials. There are plenty of youtube vedios out there you can follow to see how its created. The AUTH_SECRET is a value you provide.
Step 4: Create a simple server page under app folder.
example inside /app/comments/page.tsx
import { auth, signIn, signOut } from '@/lib/auth';
import SessionData from "@/components/session-data";
export default async function Page(){
const session = await auth();
const user = session?.user;
if (!user) {
return (
<form
action={async () => {
'use server';
await signIn('github');
}}
>
<button type="submit">Sign In</button>
</form>
);
}
return <>
<SessionData sessionFromServer={session} />
<br/>
comments
</>
}
SessionData is inside /components/session-data
import { signOut} from "@/lib/auth";
type ISessionDataProps = {
sessionFromServer: object
}
export default async function SessionData({sessionFromServer}:ISessionDataProps){
return <>
Session From Server Data: <br/>
{JSON.stringify(sessionFromServer)} <br/><br/>
<form
action={async () => {
"use server"
await signOut()
}}
className="w-full"
>
<button type={"submit"}>
Sign Out
</button>
</form>
</>
}
And thats It folk! Leave a comment if it went well. cheers.